Dynamic initialization of a variable in C++
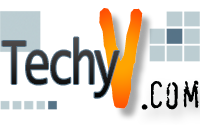
#include <iostream>
using namespace std;
Â
int main() {
 double radius = 6.0, height = 6.0;
Â
 // dynamically initialize volume
 double volume = 3.1416 * radius * radius * height;
Â
 cout << "Volume is " << volume;
Â
 return 0;
}
Â
In the sample source code above,  you can see that the variable "radius" and 'height" was just initialized first and then the values of those variables were being assigned to another variable. It is usually  used for good algorithm of a code and also for fast calculations of different variables.
Variable 'volume' should be initialized by the values of variable "radius" and "height" . Since the return value is not known until the program is actually executed, this is called dynamic initialization of variable.Â
Â
here's another example :
Â
#include <stdio.h>
int sample(void)
{
    return 20;
}
int i = sample();  //dynamic intialization
int main()
{
    printf("i = %d",i);
    return i;
}
According to the C/C++ standards global variables should be initialized before entering main(). In the above program, variable 'i' should be initialized by return value of function sample(). Since the return value is not known until the program is actually executed, this is called dynamic initialization of variable.
Â
Â
Dynamic Initialization refers to initializing a variable at runtime. If you give a C++ statement as shown below, it refers to static initialization, because you are assigning a constant to the variable which can be resolved at compile time.
Int x = 5;
Whereas, the following statement is called dynamic initialization, because it cannot be resolved at compile time.
In x = a * b;
Initializing x requires the value of a and b. So it can be resolved only during run time.
Dynamic initialization is mostly used in case of initializing C++ objects.