C++ operators and their precedence level
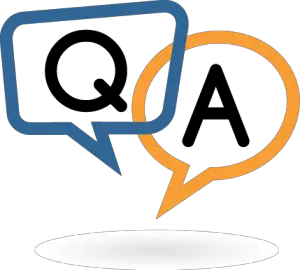
How many operators are used in C++ language?
Also describe the precedence of operators.
Write a program to show which operator has high precedence.Â
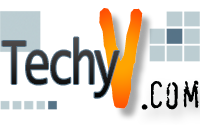
How many operators are used in C++ language?
Also describe the precedence of operators.
Write a program to show which operator has high precedence.Â
I do not have the exact number of operators in C++, but some are as follows:
Â
From most priority to lowest priority, the order is as follows:
Â
Priority Level |
Operator |
Description |
Grouping |
1 |
() [] . -> ++ — |
postfix |
Left-to-right |
2 |
++ — ~ ! |
unary (prefix) |
Right-to-left |
* & |
indirection and reference (pointers) |
||
+ – |
unary sign operator |
||
3 |
(type) |
type casting |
Right-to-left |
4 |
.* ->* |
pointer-to-member |
Left-to-right |
5 |
* / % |
multiplicative |
Left-to-right |
6 |
+ – |
additive |
Left-to-right |
7 |
<< >> |
shift |
Left-to-right |
8 |
< > <= >= |
relational |
Left-to-right |
9 |
== != |
equality |
Left-to-right |
10 |
& |
bitwise AND |
Left-to-right |
11 |
^ |
bitwise XOR |
Left-to-right |
12 |
| |
bitwise OR |
Left-to-right |
13 |
&& |
logical AND |
Left-to-right |
14 |
|| |
logical OR |
Left-to-right |
15 |
?: |
conditional |
Right-to-left |
16 |
= *= /= %= += -= >>= <<= &= ^= |= |
assignment |
Right-to-left |
17 |
, |
comma |
Left-to-right |
Â
Sample program that shows the meaning of precedence:
               #include (iostream>
               using namespace std;
              Â
               int main ()
               {
                               int a=10 ,b=20, c=30, d, e, f;
                               d=a+a*b-c;
                               e=(a*b+a)/c;
                               f=(a*(b/c));
                               cout << d << endl;
                               cout << e << endl;
                               cout << f << endl;
                               return (0);
               }
Â
The output of the program is:
Â
180
7
0
Â
In the given program:
The statement a+a*b-c; a*b is evaluated first then the operators +and – which gives the final result of 180.
In the statement (a*b+a)/c, the operators inside the parenthesis is first evaluated. The multiplication operator is evaluated before the addition operator. Then the last operator is the division which gives the final result of 7.
In the last statement parentheses are also used, the innermost expression is evaluated first giving the result of 0 then multiplied with the last variable which gives the final result of 0.
Hi Eleanor,
C++ uses operators to operate and manage its variables and constants.
You may see that C++, unlike any other languages which uses words, uses signs which are not part of the alphabet but are accessible in the keyboard. This is what makes C++ code international and shorter.
There are 55 operators all in all on C++. This includes arithmetic, comparison/relational, logical, bitwise, compound assignment, member and pointer and other operators.
To see the precedence of the operators, you can check here:
Â