Sorting algorithms form the basis for understanding and analyzing the algorithms. Sorting indicates arranging elements in ascending or descending order. It facilitates us to search the data efficiently in less time. A proper order of data is the result of sorting.
1. Quick Sort
It is considered one of the fastest sorting algorithms using divide and conquer strategy. The time complexity is n log n in the average case. We apply the conquer technique recursively for two sub-arrays. Then, we combine the already sorted array.
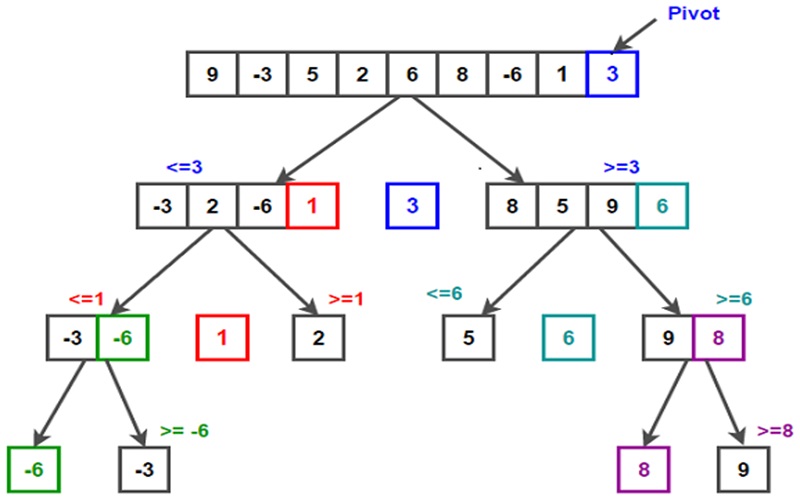
2. Merge Sort
Merge Sort is a recursive algorithm that works divides the given array into smaller arrays called sub-arrays. The sorted sub-arrays merged to form the final array that depicts the sorted array.
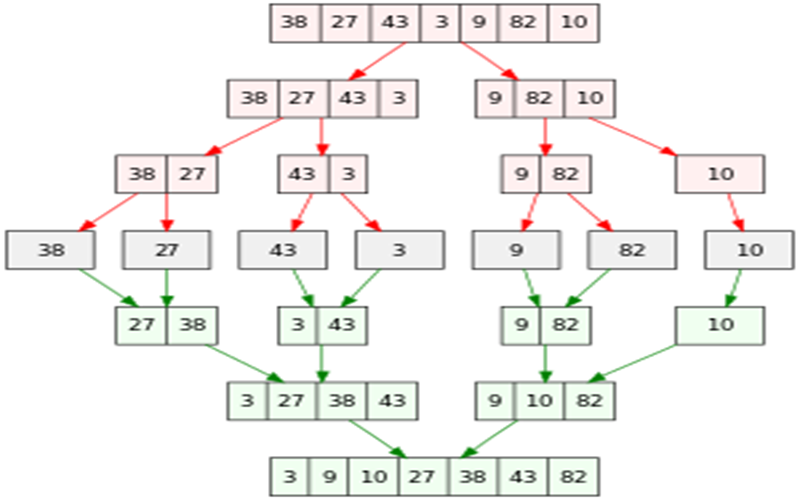
3. Heap Sort
This sorting algorithm uses a binary heap as a data structure to sort array elements. We heap a complete binary tree to make it a max heap. Even this sorting algorithm uses the recursion method.
Working of Heap Sort
The tree satisfies the Max-Heap property. The most oversized item among the elements is the root node.
Swap: Place the root element at the end of the array. We place the last item of the tree in the available place.
Remove: The heap size is reduced by one.
Heapify: Heap root element again. The most significant is the root. We repeat the above steps until all the elements are in order.
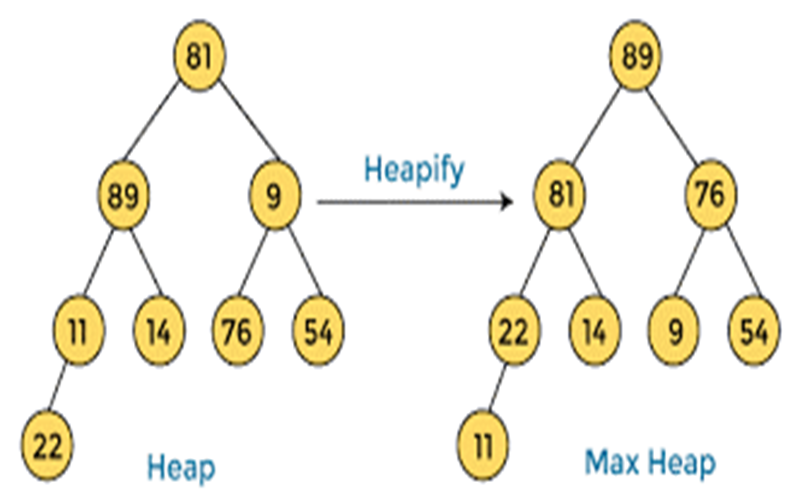
4. Insertion Sort
This sorting algorithm is in-place-comparison based on containing a sub-list. The list is sorted and inserting an element is done by finding an appropriate place to insert the element to the sub-list. It is simple and efficient for small sets of data.
Working of Insertion Sort
Step 1: When we encounter the first element, we assume the element is alread sorted.
Step 2: We store the next element as a key.
Step 3: Compare the key with the present elements in the sorted array.
Step 4: We check the element in the sorted array with the selected element. We proceed to the following element if it is smaller than the current element. We shift the elements towards the right if it is greater.
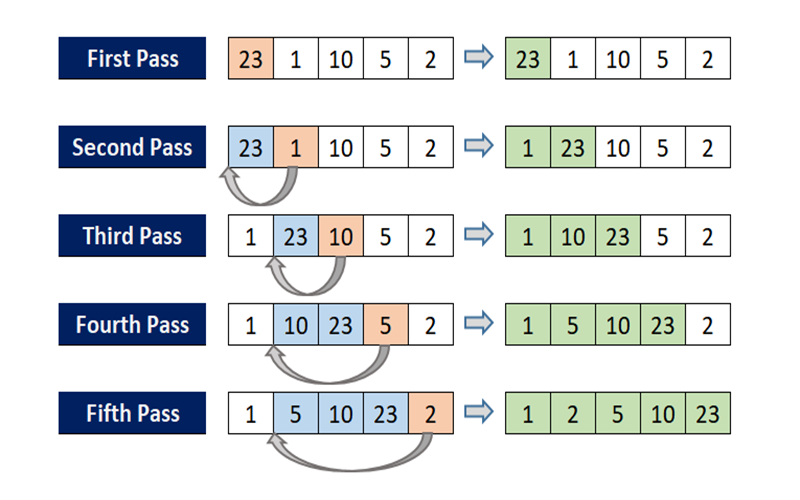
5. Selection Sort
The above algorithm is simple and easy to understand. It is not feasible for large data sets.
Steps to implement Selection Sort
Step 1: Selection: The array list is divided into sorted and unsorted lists.
Step 2: Finding minimum element: The smallest element from the unsorted array is selected. Next, we swap the selected element with the element in the first position. We repeat the steps until we get sorted ascending or descending order elements. The time complexity is worse than the insertion sort.
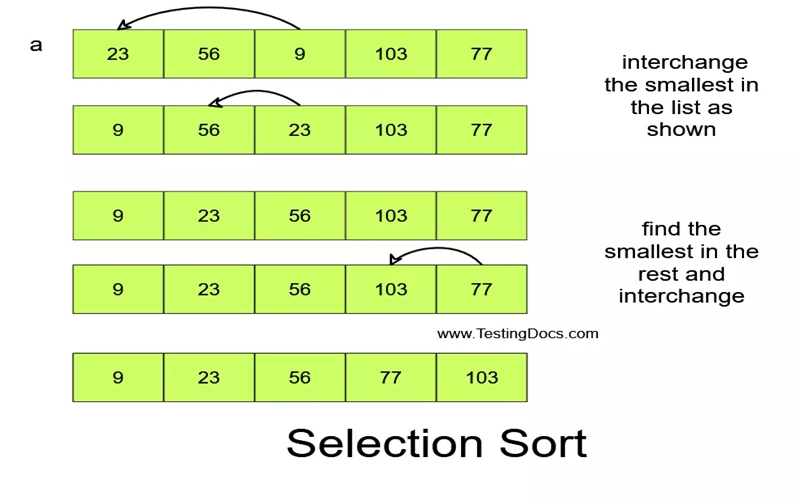
6. Bubble Sort
We know that air bubbles go to the top surface of water. Similarly, the elements sort in each iteration at the end. Hence, the name bubble sort is derived. It is an efficient sorting algorithm. First, consider an unsorted array.
Step 1: We compare the first element with the next element present.
Step 2: We swap the first element with the second element if the first element is greater than the second element present.
Step 3: We repeat the same steps until we get a sorted array.
Step 4: We observe each step movement with each iteration.
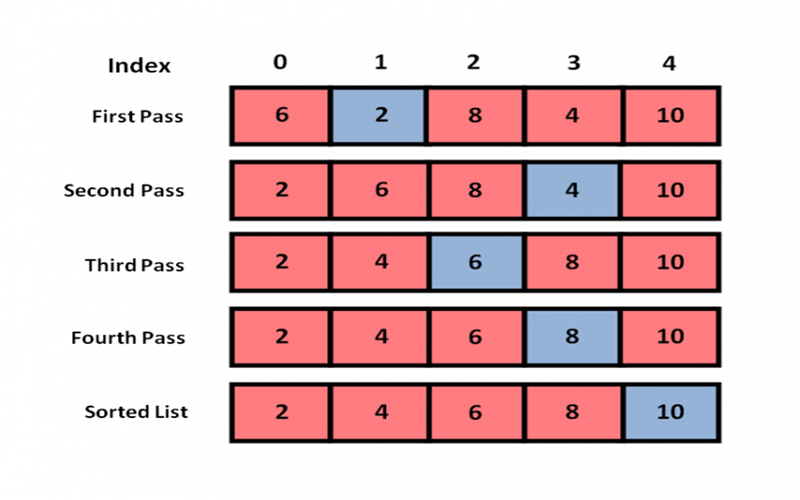
7. Radix Sort
The concept of place value plays an important role here. It is a linear sorting algorithm. This sorting technique is called bucket sorting.
Working with Radix sort
Step 1: First, we find the most significant element in the array and iterate based on the digit indexes.
Step 2: Sort the digits based on the unit place value. Then proceed to second place, hundred place, and so on.
Step 3: The array sorts itself in ascending order.
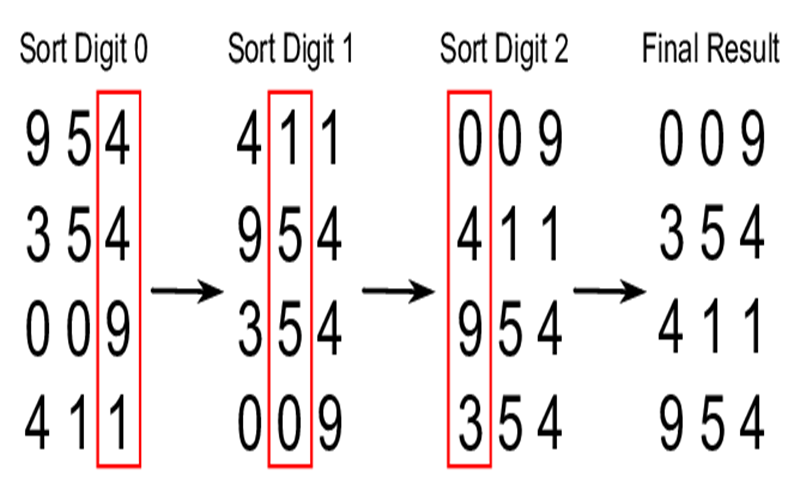
8. Counting Sort
This algorithm does not use the concept of comparison of data. It supports the negative arrangement of the array numbers.
Working
Step 1: We initialize an array and choose the maximum element from the list.
Step 2: Now, we change the array by adding the previous value and obtain a cumulative sum.
Step 3: At the end,we obtain the sorted array.
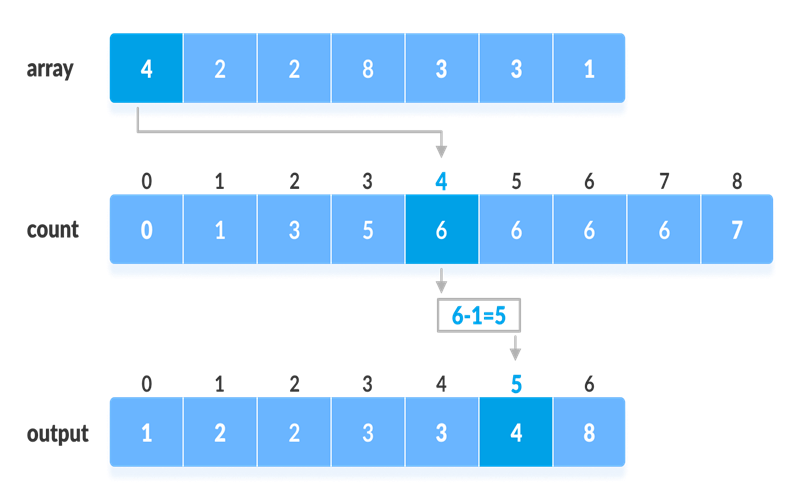
9. Bucket Sort
This sorting technique differs from the radix sort that elements are first inserted and then sorted inside a bucket.
Working procedure
Step 1: The number of buckets depends upon the range.
Step 2: Every element inserted into the bucket depends on the position.
Step 3: For each bucket, the above steps are applied.
Step 4: Concatenation of all sorted buckets.
We obtain the sorted data in a few steps for comparison.
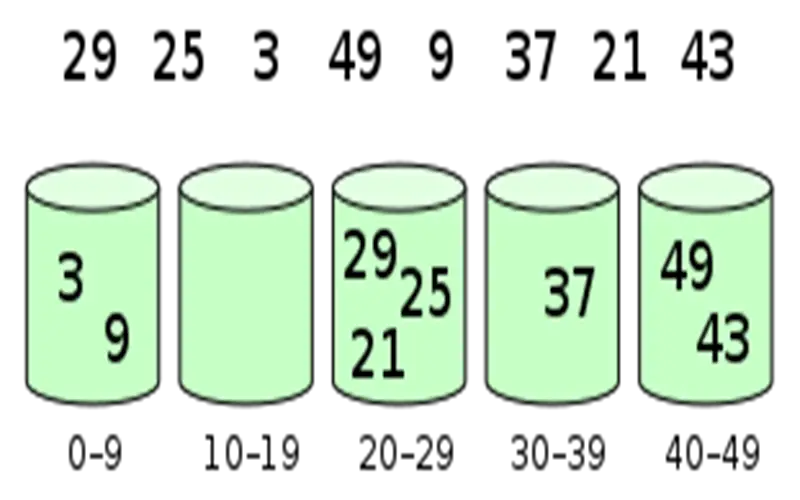
10. Tim Sort
The sort is a combination of merge sort and insertion sort. This algorithm minimizes the number of steps for comparisons.
Working
Step 1: We initialize the run size of the list.
Step 2: After we fix the run size, merge the sub-divided array.
Step 3: Continue merging till we get a sorted array.
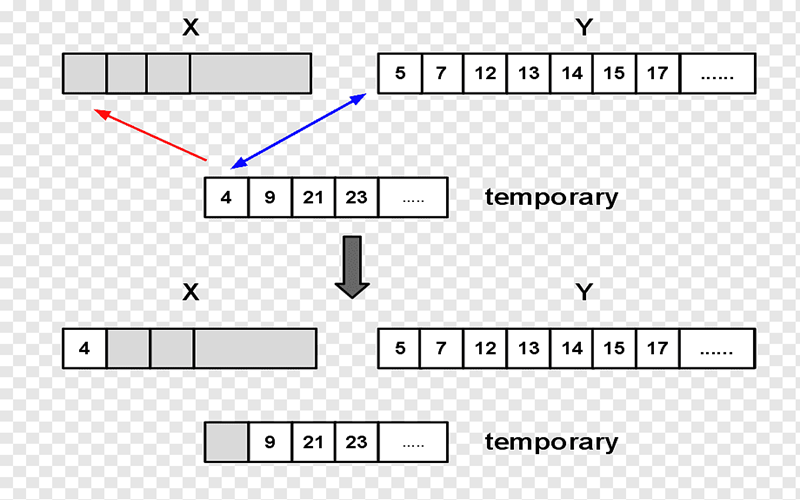
Conclusion
Each sorting algorithm has its advantages and disadvantages. Understanding and selecting the most appropriate sorting technique depends on us. The main application is in the field of data structures. It saves a lot of our time. Sorting makes arrangement of large data convenient.