Two error messages in the code while parallelizing a loop
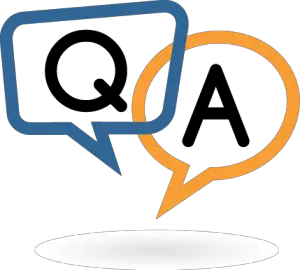
Â
Hi all,
In a class member function, I try to parallelize a loop, but I find two error messages in the code:
class myclass Â
{ Â
public: Â
int _k; Â
void f(int nb_examples, int nb_try) Â
{ Â
   int i; Â
   int ks[nb_try]; Â
   // assignment to elements in ks Â
   omp_set_num_threads(_nb_threads); Â
  #pragma omp parallel shared(ks) private(i, _k) // error: ‘myclass::_k’ is not a variable in clause ‘private’ Â
   { Â
  #pragma omp for schedule(dynamic) nowait Â
    for(i=0; i < nb_try; i ++){ Â
     _k = ks[i]; Â
     if (_k > nb_examples)  break;// error: break statement used with OpenMP for loop Â
     // operations on _k Â
    } Â
   } Â
} Â
}
I need to solve these errors. Please help me.
Thanks in advance.
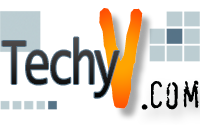