How to change the size of the array and the type?
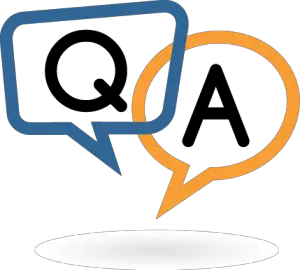
I want to implement a program to change the size of the array and the type of it too. But not using dynamic array methods. How can I do this? please help me? thanks.
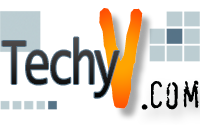
I want to implement a program to change the size of the array and the type of it too. But not using dynamic array methods. How can I do this? please help me? thanks.
I have understood your problem about changing size of an array.
I assume you have created array in C++ as hereunder.
Void main(){
int A[ ] = new int [10];
}
I must explain you a fact that as we create array in C++ using new operator, we are incorporating dynamic methods to our program.
However as we use malloc, realloc & free function found in header file #include<alloc.h> in C, to allocate, reallocate, and destroy a previously allocated array. We have operators called new & free to allocate and destroy the allocated space.
No built in operator or function in C++ does to change the size of an array.
We can Change array size by including header file #include<alloc.h> and follow instructions below.
Syntax of Allocating memory
arrayname = (type *) malloc (size_of_new_array * sizeof(type));
Just replace statement of newwith malloc statement as under
#include<alloc.h>
void main(){
int *A=(int *) malloc ( sizeof ( int ) * 3 );
}
Now you can use the dynamic array as usual.
To resize the array from 3 to 5 syntax,
arrayname = (type *) realloc (arrayname , size_of_new_array * sizeof(type));
void main(){
int *A=(int *) realloc ( A,sizeof ( int ) * 5 );
}
Infact, realloc in C is a function which does four basic operations for the reallocation.
1.    Allocate the new space for array.
2.    Copy previous array to this new array.
3.    Deallocate or free the previous array.
4.    The new array is returned as a pointer.
The Steps are pictorially and programmatically shown below.
However If you don't want to use realloc or malloc, do the following.
Try implementing the use of realloc with new operator.(Four steps above)
Void main(){
//create an array of size 3
Int *A[]=new int[3];
//Initialize the array
A[0]=1;
A[1]=2;
A[2]=3;
//recreate a new array of size 5 (Step1)
Int *B[]=new int[6];
//Copy array A to Array B.(Step2)
For(int i=0;i<2;i++){
B[i]=A[i];
}
//Two spaces B[3] and B[4] are empty.
B[3]=4;
B[4]=5;
//Hold address of A for deletion of memory space.
Int *temp=A;
//Assign address of B-array to A-array.
A=B;
//delete memory previously held by A (Step3)
Delete(temp);
//Now you Can see the new array of six values.
For(int i=0;i<4;i++){
Cout<<A[i];
}
}
You can Change the type of array in the new array and while copying data, use proper typecasting.
Dear Olivia Matthews,
Once it created it’s not possible to change array size. You have to temporally copy the old one and delete the old one you created, and make a new one and paste the old one data to new one. I hope this will work.
Thank you.