How to Convert XLS file to XML using C#
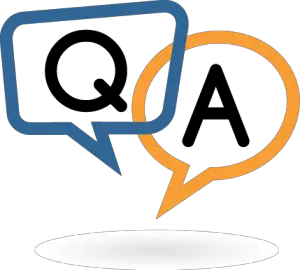
Hi all,
I want to convert an XLS file to XML file automatically.
i am doing this by using manual method like in this code.
Microsoft.Office.Interop.Excel.Sheets sheets = theWorkbook.Worksheets;
   Microsoft.Office.Interop.Excel.Worksheet worksheet = (Microsoft.Office.Interop.Excel.Worksheet)sheets.get_Item(1);
      range = worksheet.UsedRange;
               for (rcnt = 1; rcnt <= range.Rows.Count; rcnt++)
               {
                   using (XmlWriter writer = XmlWriter.Create("Demo.xml"))
                   {
                       writer.WriteStartDocument();
                       writer.WriteStartElement("Demo");
                       writer.WriteElementString("Name", (string)(range.Cells[rcnt, 1] as Microsoft.Office.Interop.Excel.Range).Value2);
                       writer.WriteElementString("Fname", (string)(range.Cells[rcnt, 2] as Microsoft.Office.Interop.Excel.Range).Value2);
                       writer.WriteElementString("Country", (string)(range.Cells[rcnt, 3] as Microsoft.Office.Interop.Excel.Range).Value2);
                       writer.WriteEndElement();
                       writer.WriteEndDocument();
                   }
               }
Is there is anyother function which can be used to easily convert file?
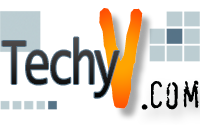