C code that terminates the program
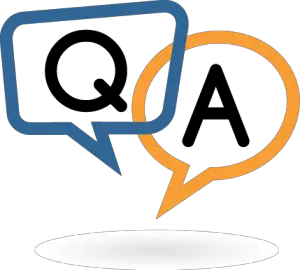
What kind of code I should use on C programing that allows the user to type a certain letter to terminate the program and does not terminate it until it is the same letter the programmer asks.?
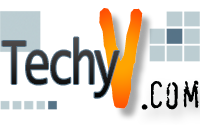
What kind of code I should use on C programing that allows the user to type a certain letter to terminate the program and does not terminate it until it is the same letter the programmer asks.?
The following code snippet asks for a character which is known for exit. and then enters a loop where it asks for characters one by one and checks for necessary exit value. If found, it exits.
#include<stdio.h>Â
#include<conio.h>
void main(){
char exitchar,newchar;
printf("Enter the character for exit.");
scanf("%c",exitchar); Â Â Â //The character to exit is given as input
while ((newchar=getch())!=exitchar){ Â Â //getch() accepts a character and first places in newchar then checks for exit.
printf("%c",newchar); Â //print on screen
}
}
You may write the equivalent codeÂ
void main(){
char exitchar,newchar;
printf("Enter the character for exit.");
scanf("%c",exitchar); Â Â Â //The character to exit is given as input
while (1){ Â Â Â
newchar=getch();
If (newchar==exitchar)
return(0); Â Â Â //If the exitcharacter is found.
else
printf("%c",newchar); Â //print on screen
}
}
Hello Ulysses Arredonto,
These are the working snippets for your query which shows the requirements you desire.
Try these and sort your program as you want.
#include<conio.h>
#include<stdio.h>
void main(void) {
   char e,n;
   printf ("Enter the character for exit: ");
   scanf ("%c", e);               // here e stores the exit character
   while ((n=getch())!=e) {         // the value is stored in n by getch() and checks for the exit value
       printf ("%c",n);            // values are shown on the screen until the e entered
   }
}
This is the code in C++ environment which might be helpful to you …
#include<conio.h>
#include<iostream.h>
#include<stdio.h>
void main(void) {
   char e,n;
   cout << "Enter the character for exit: ";
   cin >> e;               // here e stores the exit character
   while ((n=getch())!=e) {   // the value is stored in n by getch() and checks for the exit value
       cout << n;            // values are shown on the screen until the e entered
   }
}
Hope this will help you in your problem.
Thank you
Emon Asejo
I tried this and it worked.
#include<conio.h>
#include<stdio.h>
void main(void) {
   char e,n;
   printf ("Enter the character for exit: ");
   scanf ("%c", e);               // here e stores the exit character
   while ((n=getch())!=e) {         // the value is stored in n by getch() and checks for the exit value
       printf ("%c",n);            // values are shown on the screen until the e entered
   }
}
This is the code in C++ environment which might be helpful to you …
#include<conio.h>
#include<iostream.h>
#include<stdio.h>
void main(void) {
   char e,n;
   cout << "Enter the character for exit: ";
   cin >> e;               // here e stores the exit character
   while ((n=getch())!=e) {   // the value is stored in n by getch() and checks for the exit value
       cout << n;            // values are shown on the screen until the e entered
   }
}
THANK YOU FOR THE HELP!!