How to resize encoded videos using C#?
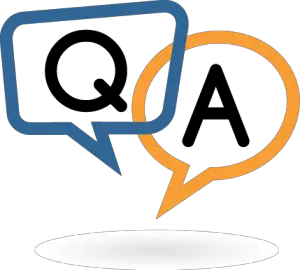
I'd like to know how to resize encoded video on C#. Can you teach me how to deal with it. I need your big help on this.
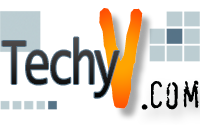
I'd like to know how to resize encoded video on C#. Can you teach me how to deal with it. I need your big help on this.
Try below code :
MP4OutputFormat mp4Format = new MP4OutputFormat();
mp4Format.VideoProfile = new Microsoft.Expression.Encoder.Profiles.HighH264VideoProfile();
mp4Format.AudioProfile = new Microsoft.Expression.Encoder.Profiles.AacAudioProfile();
mp4Format.VideoProfile.AspectRatio = new System.Windows.Size(16, 9);
mp4Format.VideoProfile.AutoFit = true;
mp4Format.VideoProfile.Size = new System.Drawing.Size(1920, 1080);
mp4Format.VideoProfile.Bitrate = new Microsoft.Expression.Encoder.Profiles.VariableUnconstrainedBitrate(6000);
mediaItem.VideoResizeMode = VideoResizeMode.Letterbox;
mediaItem.OutputFormat = mp4Format;
Hello Lawsonn Henry,
Hope that my message finds you well.
You can use the following steps:
Step No1: Create a new Job in C# as the following:
Job X = new Job();
You can name the Job whatever you want, in the example above we named it “X”.
Step No 2: Create Media Item with the path of your file as the following:
MediaItem CreateMediaItem = new MediaItem(TheFilePath);
You can name the Media Item whatever you want, in the example above we named it “CreateMediaItem”.
Also you should replace “TheFilePath” with the file path that you want.
Step No 3: Declare an integer that will retrieve the size of the video as the following:
Int GetSize = mediaItem.OriginalVideoSize();
We name the integer “GetSize” and you can replace it with whatever you want.
Step No 4: Create cases from the size of the video using “If statement” as the following:
If (GetSize.Width <= 1920 && GetSize > 1080)
{
Code will be here
}
Else if (GetSize.Width <= 1080 && GetSize > 900)
{
Code will be here
}
….etc
Step No 5: Inside each If statement you will do the following:
– Declare MP4 output format:
MP4OutputFormat MyMP4OutputFormat = new MP4OutputFormat ();
Note: You can name it whatever you want.
– Connect the output format profile with the Encoder profile:
MyMP4OutputFormat.VideoProfile = new Microsoft.Expression.Encoder.Profiles.HighH264VideoProfile();
– Connect the Audio profile with the Encoder audio profile:
MyMP4OutputFormat.AudioProfile = new Microsoft.Expression.Encoder.Profiles.AacAudioProfile
– Declare the aspect ratio of the video:
MyMP4OutputFormat.VideoProfile.AspectRatio = new System.Windows.Size(16, 9);
– Make the Video profile auto fit:
MyMP4OutputFormat.VideoProfile.AutoFit = true;
– Inside each (if statement) you need to put video profile size:
MyMP4OutputFormat.VideoProfile.Size = new System.Drawing.Size(1920, 1080);
– Declare the “Bitrate” for the video profile:
MyMP4OutputFormat.VideoProfile.Bitrate = new Microsoft.Expression.Encoder.Profiles.VariableUnconstrainedBitrate(6000);
– Make the Video resize mode:
CreateMediaItem.VideoResizeMode = VideoResizeMode.Letterbox;
You can choose whatever you want from the VideoResizeMode function.
– Make the output format for the video:
CreateMediaItem.OutputFormat = mp4Format;
– Name the output file:
CreateMediaItem.OutputFileName = NewFile;
– Connect the Job with the media Item:
X.MediaItems.Add(mediaItem);
Step No 6: After you finish all the cases of video size you still need to do the following steps:
– Create Sub folder for the job:
X.CreateSubfolder = false;
– Make the output directory:
X.OutputDirectory=OutputDir;
– Make the Encode for the job:
X.Encode();
– Finally Dispose the job:
X.Dispose();
Hope this solution will help you.
Kind regards,